In this tutorial, you will learn how to get the data between two dates in laravel.
In this article, I will show you how to get the data between dates using whereBetween()
, where()
and whereDate()
in Laravel.
Table of Contents
Assumption:
We will be using users
as the example for this article, we will get the users that registered or created between dates based on the created_at
column And we will get them using whereBetween()
, where()
and whereDate()
.
Let’s get started!!!
Step 1: Install Laravel
The first step is to install the Laravel, I am installing the Laravel in the get_date_beween
folder.
composer create-project --prefer-dist laravel/laravel get_date_beween
Step 3: Setup DB Credentials and Migrate
I have updated my env
file with database credentials and run the migration by using:
php artisan migrate
Step 4: Add dummy users with created date
I have added 2 users as the dummy with the created_date
.
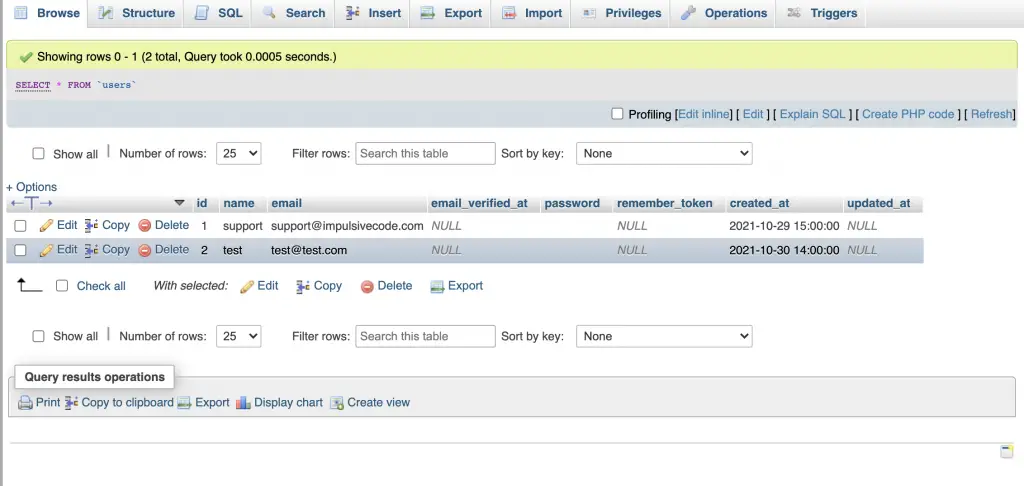
Step 5: Create Controller
Now we will create UserController
by using the following command:
php artisan make:Controller UserController
Step 6: Get data between two dates:
Let’s discuss now all three ways whereBetween()
, where()
and whereDate()
to get the data between dates in laravel.
A) Get the data between two dates using whereBetween():
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Carbon\Carbon;
use Illuminate\Http\Request;
class UserController extends Controller
{
//
public function example_1(){
$example_1=[];
//time sensitive
$start_date=Carbon::createFromFormat('d/m/Y H:i:s', '29/10/2021 15:00:00'); //gives 2021-10-29 15:00:00
$end_date=Carbon::createFromFormat('d/m/Y H:i:s', '30/10/2021 14:00:00'); //gives 2021-10-30 14:00:00
//time insensitive (not to take time)
$start_date=Carbon::createFromFormat('d/m/Y', '29/10/2021')->toDateString(); //gives 2021-10-29
$end_date=Carbon::createFromFormat('d/m/Y', '30/10/2021')->toDateString(); //gives 2021-10-30
//php time insensitive (not to take time)
$start_date=date('Y-m-d', strtotime('2021-10-29')); //gives 2021-10-29
$end_date=date('Y-m-d', strtotime('2021-10-30')); //gives 2021-10-30
$all_users=User::whereBetween('created_at',[$start_date,$end_date])
->get();
$example_1=[
$start_date,
$end_date,
$all_users,
];
echo json_encode($example_1);
}
We will be using Carbon
the library in order to get our date from d/m/Y h:m:s
converted into database timestamp using carbon function createFromFormat
.
The createFromFormat
by default takes time, if you don’t want time you can use ->toDateString()
to ignore the time or you can use PHP date
and strtotime
function. Then in that case whereBetween
will consider time as 00:00:00
.
WhereBetween
takes two array elements as the start_date
and end_date
on the column name which in our case is created_at
.
Output:
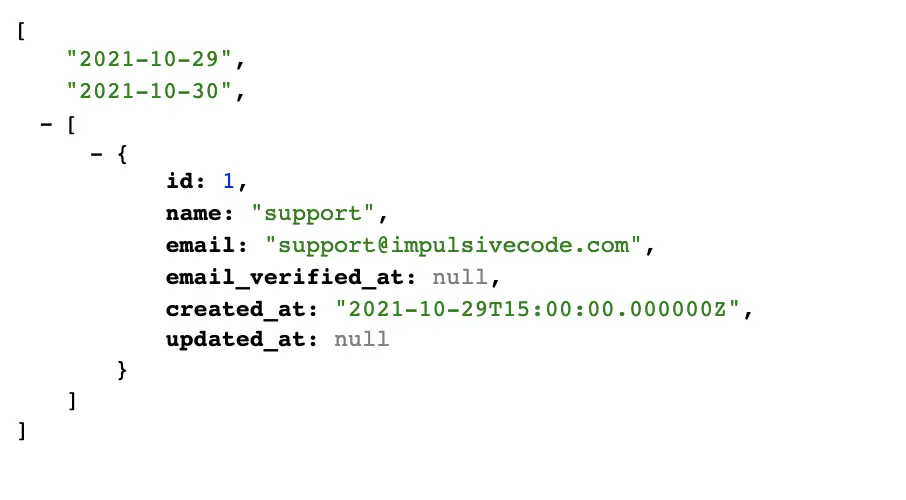
It will give one user only as because other user time is greater than 2021-10-30 00:00:00
.
2. Get the data between two dates using where():
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Carbon\Carbon;
use Illuminate\Http\Request;
class UserController extends Controller
{
//
public function example_2(){
//example 2
//--time sensitive
$start_date=Carbon::createFromFormat('d/m/Y H:i:s', '29/10/2021 15:00:00'); //gives 2021-10-29 15:00:00
$end_date=Carbon::createFromFormat('d/m/Y H:i:s', '30/10/2021 14:00:00'); //gives 2021-10-30 14:00:00
//--time insensitive (not to take time)
$start_date=Carbon::createFromFormat('d/m/Y', '29/10/2021')->toDateString(); //gives 2021-10-29
$end_date=Carbon::createFromFormat('d/m/Y', '30/10/2021')->toDateString(); //gives 2021-10-30
//--php time insensitive (not to take time)
$start_date=date('Y-m-d', strtotime('2021-10-29')); //gives 2021-10-29
$end_date=date('Y-m-d', strtotime('2021-10-30')); //gives 2021-10-30
$all_users=User::where('created_at','>=',$start_date)
->where('created_at','<=',$end_date)
->get();
$example_2=[
$start_date,
$end_date,
$all_users,
];
echo json_encode($example_2);
}
Same as the previous example we are using Carbon
the library to get converted from d/m/Y h:m:s
to YYYY-MM-DD HH:MM:SS
using carbon function createFromFormat
.
If you don’t want time in createFromFormat
add the function toDateString()
or use the php date
and strtotime
function. Then in that case where
will consider time as 00:00:00
.
In Where
, we are adding two conditions that the date should be greater than equal to start_date
and less than equal to end_date
.
The output of this is the same as whereBetween()
, as both are same.
Output:
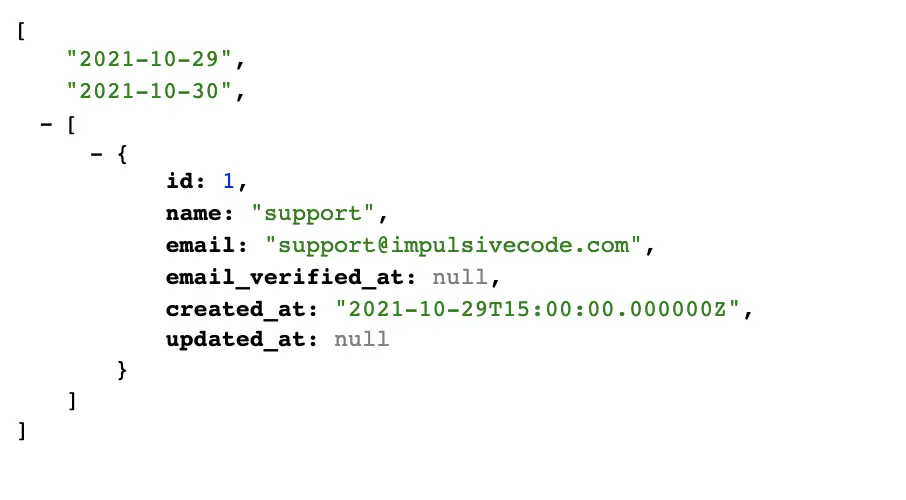
Same output as whereBetween()
, one user returned only as because other user time is greater than 2021-10-30 00:00:00
.
3. Get the data between two dates using whereDate():
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Carbon\Carbon;
use Illuminate\Http\Request;
class UserController extends Controller
{
//
public function example_3(){
//example 3
//--time sensitive
$start_date=Carbon::createFromFormat('d/m/Y H:i:s', '29/10/2021 15:00:00'); //gives 2021-10-29 15:00:00
$end_date=Carbon::createFromFormat('d/m/Y H:i:s', '30/10/2021 14:00:00'); //gives 2021-10-30 14:00:00
//--whereDate is time insensitive, where you pass or not it will not take time
$all_users=User::whereDate('created_at','>=',$start_date)
->whereDate('created_at','<=',$end_date)
->get();
$example_3=[
$start_date,
$end_date,
$all_users,
];
echo json_encode($example_3);
}
In the above example, we are using whereDate()
have similar where()
condition, in which we are passing two conditions that the date should be greater than equal to start_date
and less than equal to end_date
.
whereDate()
is time insensitive, where you pass or not it will not take the time.
Output:
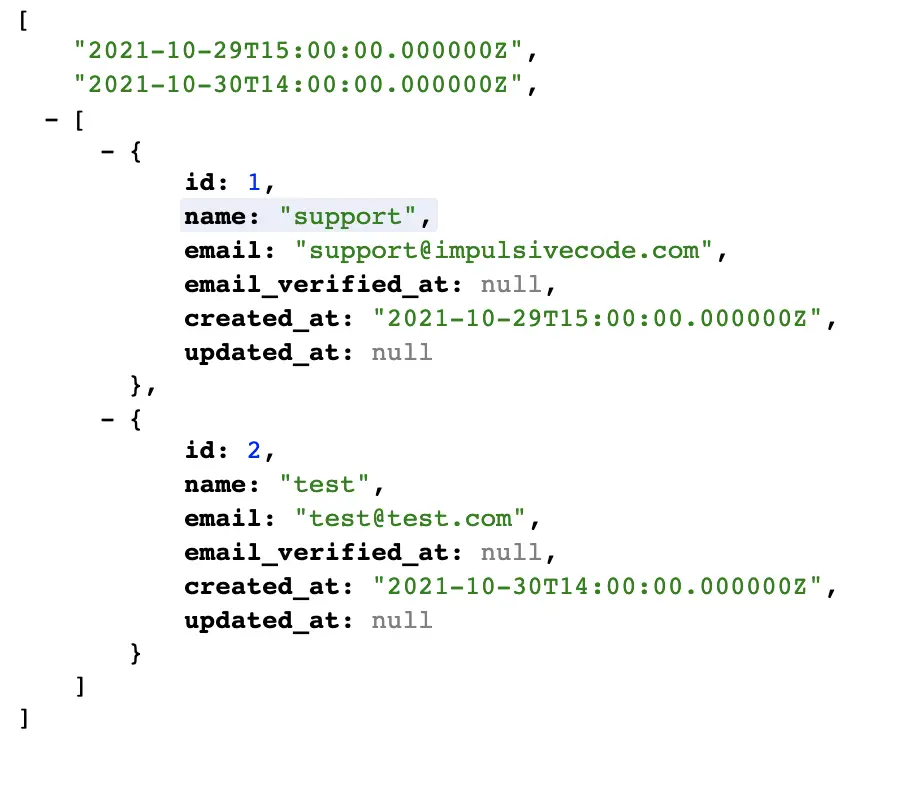
It will give us two records, as because whereDate()
doesn’t respect time at all.
Conclusion:
Hope you have learned today, how to get records between dates using whereBetween()
, where()
and whereDate()
using Laravel.
Let me know if you any questions in comments!
I will see you in the next one!
Write a Reply or Comment