Hey guys, in this tutorial I am going to cover how you can create the pagination in laravel 10 with the example of bootstrap 5.
This is a step-by-step guide on laravel pagination example with Bootstrap 5 which is easy to follow. In this article, we are using articles as the example case and will paginate the articles.
We will start off with setting up the laravel, then updating the DB credentials, creating model, migration and controller, connecting route with controller and creating the view, getting data from database and using paginate
and links
to create the pagination.
So let’s get started.
Table of Contents
Step 1: Install Laravel
First of all, you need to install the laravel. I am installing the laravel in pagination folder.
composer create-project --prefer-dist laravel/laravel pagination
Step 2: Update the ENV file and start server
In this step, you need to update the DB credentials in the env
file and start the server by typing:
php artisan serve
Step 3: Create Article model and migration
Now we will create the article model and its migration. For migration, we are using -m
flag.
php artisan make:model article -m
Step 4: Update the migrate function and run migration
We will add the title
and description
in the article migration up
function in database/migrations/create_articles_table.php
.
Schema::create('articles', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->string('description');
$table->timestamps();
});
Now we need to migrate the table we created.
php artisan migrate
Step 5: Add the fake article data
You can skip this step if will add the data manually in the table.
To add the dummy data we are using the seeders, so open up the seeders/DatabaseSeeder.php
and update the run
function to have the following code:
$faker= Faker::create();
foreach (range(1,100) as $index){
Article::create([
'title'=>$faker->sentence(6),
'description'=>$faker->paragraph(1),
]);
}
We are using Faker
to add the dummy title and description in the Article
Table. 'title'=>$faker->sentence(6)
will add the 6 words as the title and 'description'=>$faker->paragraph(1)
will add 1 sentence as the description. We are adding 100 articles by doing that.
Make sure to include the use App\Models\Article;
and use Faker\Factory as Faker;
in the header as well.
To generate the data run command:
php artisan db:seed
Step 6: Create Controller and Route
Run the following command to create ArticleController
.
php artisan make:controller ArticleController
Place the following code in the ArticleController
.
<?php
namespace App\Http\Controllers;
use App\Models\Article;
use Illuminate\Http\Request;
class ArticleController extends Controller
{
//
public function get_data(){
$articles = Article::all();
return view('home', compact('articles'));
}
}
Now lets create the route in routes/web.php
file.
Route::get('get_data',[\App\Http\Controllers\ArticleController::class,'get_data']);
Step 7: Create View
Now create a blade file resources/views/home.blade.php
and place the following code:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css">
</head>
<body>
<div class="container mt-5">
<h1 class="text-center">All the Articles</h1>
<div class="row mt-5">
@foreach($articles as $each_article)
<div class="col-lg-4">
<div class="card mt-2">
<div class="card-body">
<h5 class="card-title">{{$each_article->title}}</h5>
<p class="card-text">{{$each_article->description}}</p>
</div>
</div>
</div>
@endforeach
</div>
</div>
</body>
</html>
In this above home
blade template, we have included the bootstrap and have used the card components to create the layout to show all the articles by traversing the $articles
variable which we have passed from the ArticleController
.
If you open the browser and type get_data
with the url on which your application is running you will see something like this.
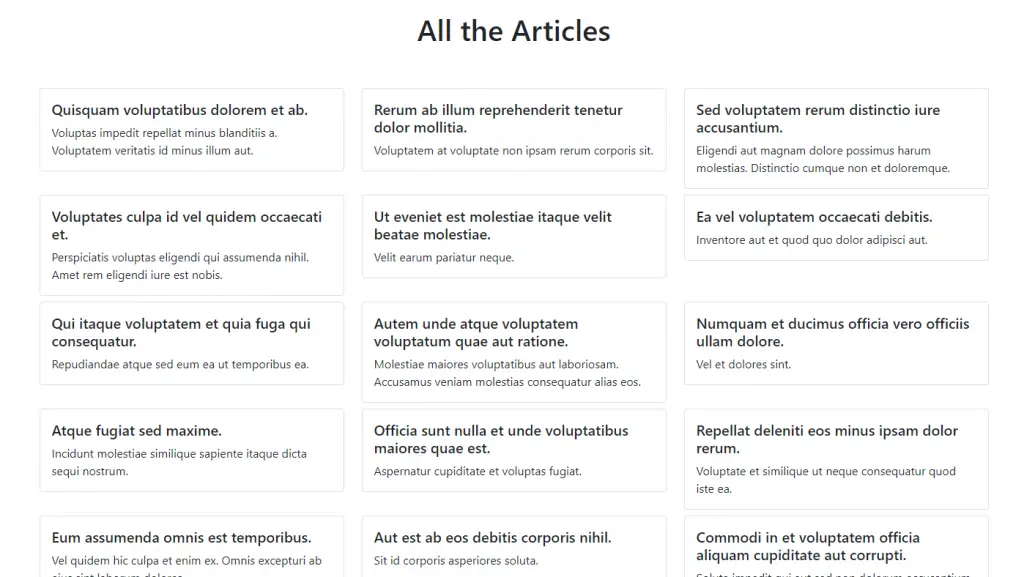
So at this point the articles are loading without any pagination. Lets convert it so that it will load with pagination.
Step 8: Add the paginate in Controller
So in order to show articles with pagination we need to replace Article::all();
to Article::paginate(10);
in ArticleController
.
So our final controller code will look like:
<?php
namespace App\Http\Controllers;
use App\Models\Article;
use Illuminate\Http\Request;
class ArticleController extends Controller
{
//
public function get_data(){
$articles = Article::paginate(10);
return view('home', compact('articles'));
}
}
Where 10
is number of articles we want to display per page. You can change this number as you like. For now I am sticking to 10
articles per page.
Now if you refresh the browser page you will see that only 10
articles are loading but there is no pagination.
So next step is we need to add the links in the home.blade.php file as well.
Step 9: Add the pagination links in the view file
So in the resources/views/home.blade.php
, after the row div, we need to add {!! $articles->links() !!}
.
So our final view file will look like this:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css">
</head>
<body>
<div class="container mt-5">
<h1 class="text-center">All the Articles</h1>
<div class="row mt-5">
@foreach($articles as $each_article)
<div class="col-lg-4">
<div class="card mt-2">
<div class="card-body">
<h5 class="card-title">{{$each_article->title}}</h5>
<p class="card-text">{{$each_article->description}}</p>
</div>
</div>
</div>
@endforeach
</div>
{!! $articles->links() !!}
</div>
</body>
</html>
So the final result will look like this:
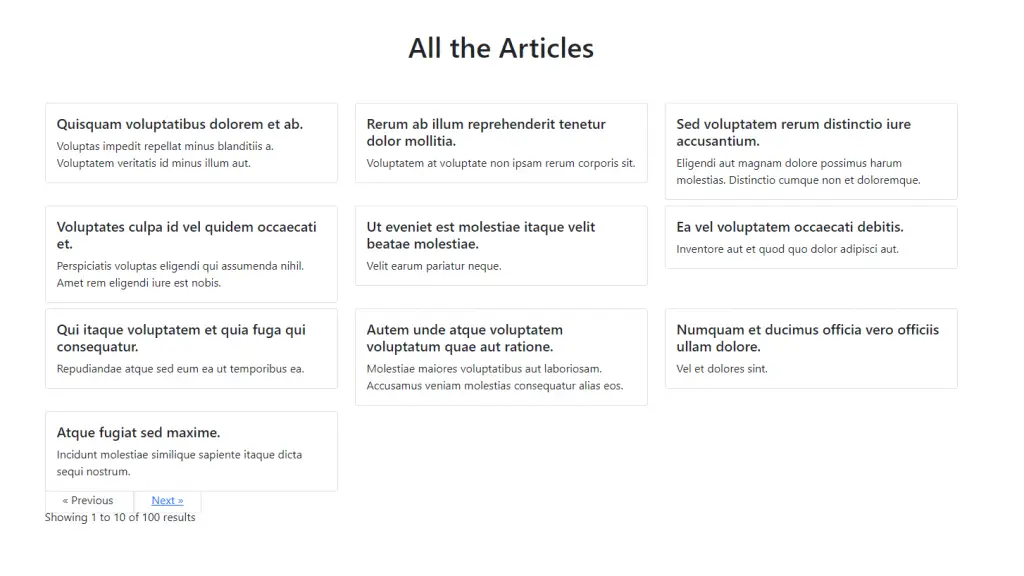
As you can see that the pagination links but its not looking good. As there is no CSS for the pagination. You can either write custom css for that or you can use the bootstrap
pagination.
In order to use the bootstrap
pagination, we need to add the Paginator::useBootstrap();
in boot()
function in app/Providers/AppServiceProvider.php
.
So your AppServiceProvider
file will look like this:
<?php
namespace App\Providers;
use Illuminate\Pagination\Paginator;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
//
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
//
Paginator::useBootstrap();
}
}
And if you refresh the page, it will look like this:
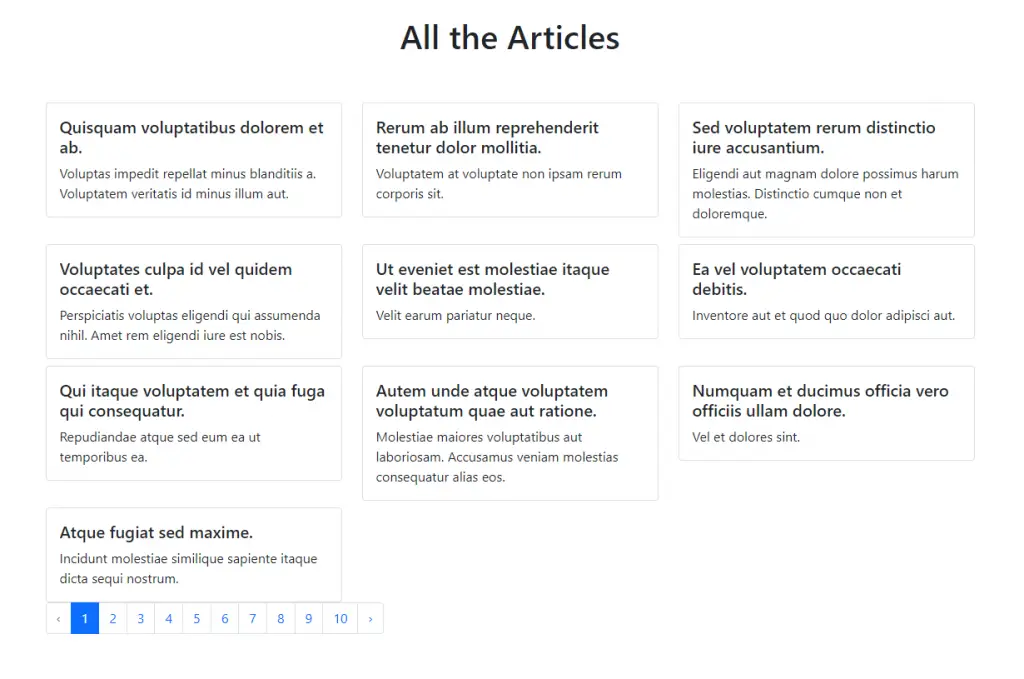
Okay we are finally here just add the following CSS
style in the head of the home.blade.php
file.
<style>
.pagination{
float: right;
margin-top: 10px;
}
</style>
So the final home.blade.php
will look like this:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css">
<style>
.pagination{
float: right;
margin-top: 10px;
}
</style>
</head>
<body>
<div class="container mt-5">
<h1 class="text-center">All the Articles</h1>
<div class="row mt-5">
@foreach($articles as $each_article)
<div class="col-lg-4">
<div class="card mt-2">
<div class="card-body">
<h5 class="card-title">{{$each_article->title}}</h5>
<p class="card-text">{{$each_article->description}}</p>
</div>
</div>
</div>
@endforeach
</div>
{!! $articles->links() !!}
</div>
</body>
</html>
And if you refresh the browser it will look like this:
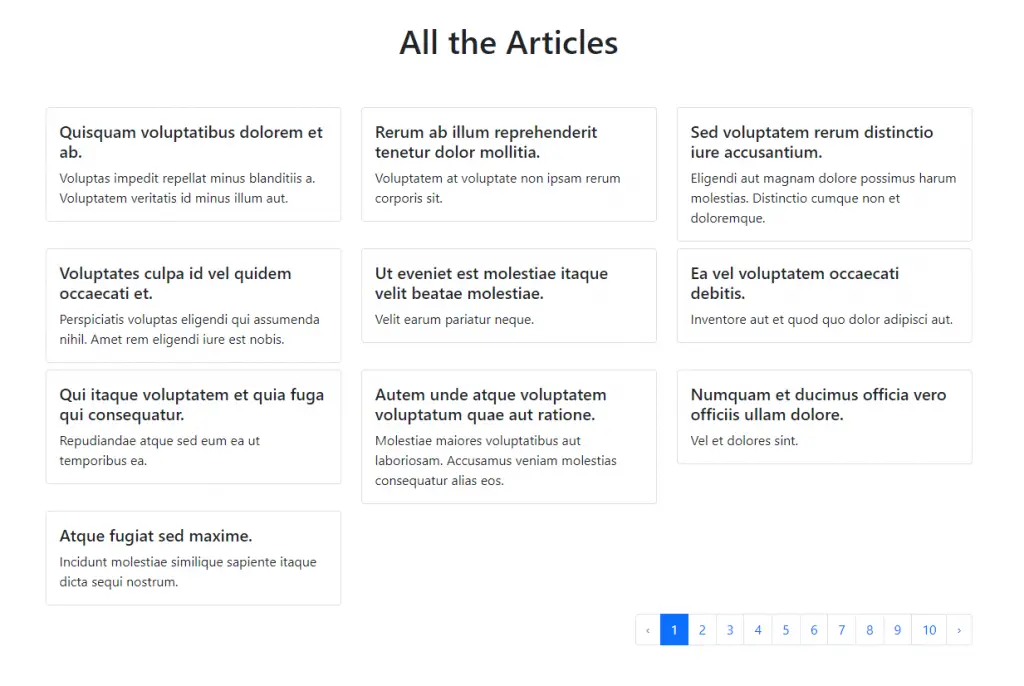
Great 🙂 That’s it.
Conclusion
Awesome, you have just finished the tutorial on Laravel 8 pagination with Bootstrap 4. Hope you have learned something new today.
Let me know if you face any problems in the comments.
See you again in another tutorial 🙂
Write a Reply or Comment