In this post, we will see how you can validate the password and confirm the password using Jquery.
First, we will create the bootstrap form, then we will use the keyup
event in order to validate the password and confirm the password in jquery.
Let’s get started.
Table of Contents
Step 1: Include bootstrap and jquery
First I will include the bootstrap and jquery.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Validate Jquery Password and confirm password</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
</body>
</html>
Step 2: Add the form
Then in the body tag, I have created the Bootstrap form in which I have email, password and confirm password fields. Then I have given id="password"
to the password field and id="confirmpassword"
to the confirm password field.
I have also added div with confirm-message
class so that we can show success or error message based on the input in password and confirm password fields.
<h3 class="text-center mt-5">Validate Jquery Password and confirm password</h3>
<div class="container">
<div class="row justify-content-center mt-5">
<div class="col-md-4">
<div class="mb-3">
<label for="exampleInputEmail1" class="form-label">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp">
<div id="emailHelp" class="form-text">We'll never share your email with anyone else.</div>
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="password">
</div>
<div class="mb-3">
<label for="confirmpassword" class="form-label">Confirm Password</label>
<input type="password" class="form-control" id="confirmpassword">
<div class="form-text confirm-message"></div>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</div>
</div>
Step 3: Add the jquery code
Then I have added the jquery code. In the following code, first we added keyup
event on the password
field and confirmpassword
field.
After that, I am removing the error-message
and success-message
class from confirm-message
as when we key is pressed there should be no classes.
Then I am getting values of password
and confirmpassword
field.
After that, we have checking password
field, if its empty, we are adding “Password Field cannot be empty” text to the confirm-message
and adding the error-message
to it.
Same we are doing for the confirmpassword
.
After that, we are checking if password
and confirmpassword
are same, then we are adding “Password Match!” text and success-message
class to the confirm-message
div.
If they are not the same, we are adding “Password Doesn’t Match!” text and adding error-message
class to the confirm-message
.
<script>
$('#password, #confirmpassword').on('keyup', function(){
$('.confirm-message').removeClass('success-message').removeClass('error-message');
let password=$('#password').val();
let confirm_password=$('#confirmpassword').val();
if(password===""){
$('.confirm-message').text("Password Field cannot be empty").addClass('error-message');
}
else if(confirm_password===""){
$('.confirm-message').text("Confirm Password Field cannot be empty").addClass('error-message');
}
else if(confirm_password===password)
{
$('.confirm-message').text('Password Match!').addClass('success-message');
}
else{
$('.confirm-message').text("Password Doesn't Match!").addClass('error-message');
}
});
</script>
Step 4: Add some CSS
To add the some colors I am adding some CSS to the success-message
and error-message
class.
<style>
.success-message{
color:green
}
.error-message{
color:red;
}
</style>
Here is what the final code will look like:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Validate Jquery Password and confirm password</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet">
<style>
.success-message{
color:green
}
.error-message{
color:red;
}
</style>
</head>
<body>
<form>
<h3 class="text-center mt-5">Validate Jquery Password and confirm password</h3>
<div class="container">
<div class="row justify-content-center mt-5">
<div class="col-md-4">
<div class="mb-3">
<label for="exampleInputEmail1" class="form-label">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp">
<div id="emailHelp" class="form-text">We'll never share your email with anyone else.</div>
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="password">
</div>
<div class="mb-3">
<label for="confirmpassword" class="form-label">Confirm Password</label>
<input type="password" class="form-control" id="confirmpassword">
<div class="form-text confirm-message"></div>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</div>
</div>
</form>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$('#password, #confirmpassword').on('keyup', function(){
$('.confirm-message').removeClass('success-message').removeClass('error-message');
let password=$('#password').val();
let confirm_password=$('#confirmpassword').val();
if(password===""){
$('.confirm-message').text("Password Field cannot be empty").addClass('error-message');
}
else if(confirm_password===""){
$('.confirm-message').text("Confirm Password Field cannot be empty").addClass('error-message');
}
else if(confirm_password===password)
{
$('.confirm-message').text('Password Match!').addClass('success-message');
}
else{
$('.confirm-message').text("Password Doesn't Match!").addClass('error-message');
}
});
</script>
</body>
</html>
Take a look how it will show:
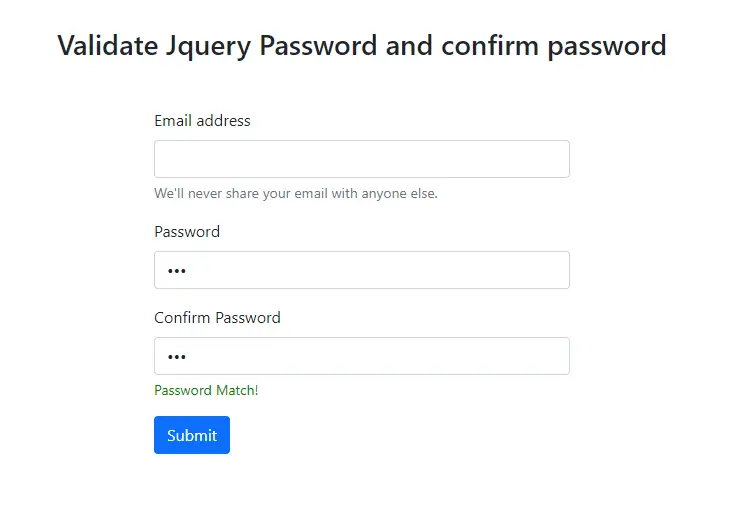
Conclusion:
Great! You have just finished the article on validate the password and confirm password using Jquery.
Let me know if you have any questions in the comments.
With that said, I am signing off.
Peace!!! Keep Learning 🙂
Write a Reply or Comment